10 Essential HTML Tags Every Web Developer Must Know
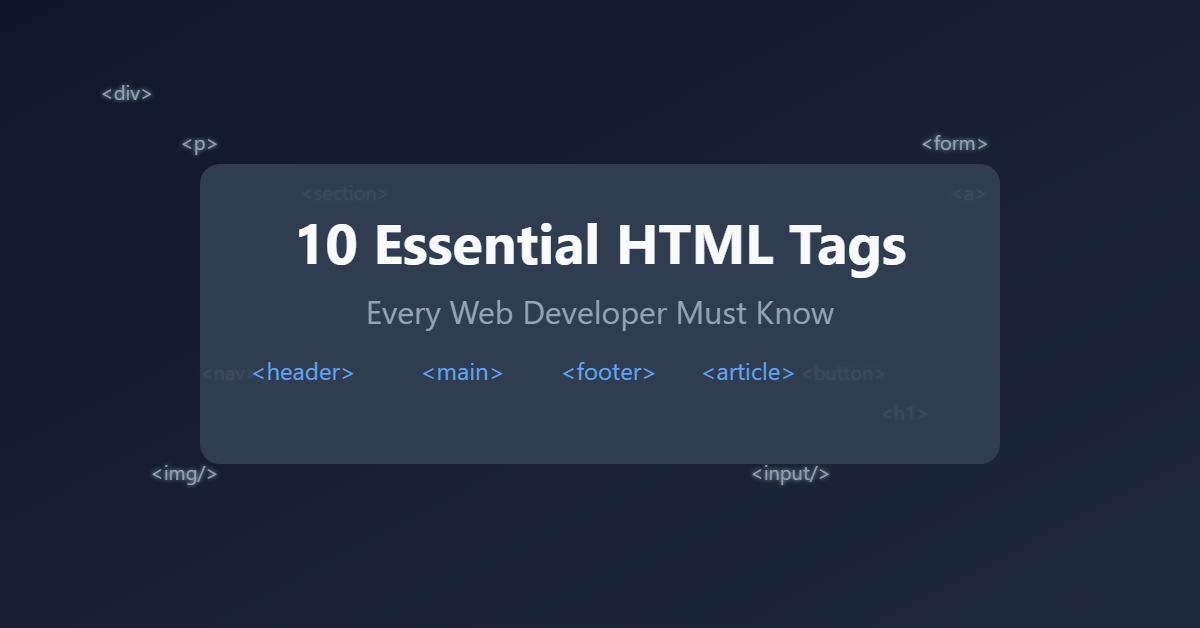
Understanding essential HTML tags is crucial for any web developer looking to create well-structured, accessible websites. Whether you’re just starting your coding journey or need a refresher, these fundamental building blocks will help you craft better web pages.
Key Takeaways
- Master the basic document structure tags (DOCTYPE, html, head, body)
- Learn semantic heading tags (h1-h6) for proper content hierarchy
- Understand container elements like div and span for layout control
- Get comfortable with interactive elements (forms, buttons, links)
- Focus on accessibility with proper alt text and ARIA attributes
- Practice combining tags to create complex layouts
- Follow HTML best practices for clean, maintainable code
Table of Contents
1. The HTML and Document Structure Tags
Let’s start with the foundation! Every HTML document needs these structural elements:
<!DOCTYPE html>
<html>
<head>
<!-- Document metadata goes here -->
</head>
<body>
<!-- Your content goes here -->
</body>
</html>
These tags create the basic structure that every webpage needs. Think of them as the skeleton of your document! If you’d like to explore all HTML tags in more detail, check out the HTML Reference Guide on MDN Web Docs.
2. Heading Tags (<h1> to <h6>)
Headers are like the chapter titles of your content. The <h1>
tag is your main title, and you can use <h2>
through <h6>
for subheadings. Here’s a pro tip: always maintain proper hierarchy – it’s great for both SEO and accessibility!
<h1>Main Title of the Website</h1>
<h2>Important Section</h2>
<h3>Subsection</h3>
<h4>Deeper Level</h4>
<h5>Even More Specific</h5>
<h6>Most Specific Level</h6>
3. The Paragraph Tag (<p>)
This might seem basic, but the humble <p>
tag is your bread and butter for text content. It automatically adds some spacing around your text blocks, making your content much more readable. No more walls of text!
<p>This is a paragraph with some text. It automatically adds spacing before and after the content.</p>
<p>This is another paragraph. Notice how it's separated from the first one!</p>
<p>You can also include <strong>bold text</strong> and <em>italic text</em> within paragraphs.</p>
4. The Link Tag (<a>)
Want to connect your pages or link to other websites? The anchor tag is your best friend:
<!-- External link -->
<a href="https://www.example.com" target="_blank">Visit Example.com</a>
<!-- Internal page link -->
<a href="/about">About Us</a>
<!-- Email link -->
<a href="mailto:[email protected]">Email Us</a>
<!-- Phone link -->
<a href="tel:+1234567890">Call Us</a>
<!-- Link with image -->
<a href="https://www.example.com">
<img src="button.jpg" alt="Click Here">
</a>
Remember to use descriptive text in your links – “click here” isn’t very helpful for accessibility or SEO.
5. Image Tag (<img>)
Pictures are worth a thousand words, right? The image tag lets you add visual content to your pages:
<!-- Basic image -->
<img src="puppy.jpg" alt="Cute puppy playing with a ball">
<!-- Image with specific dimensions -->
<img src="logo.png" alt="Company Logo" width="200" height="100">
<!-- Responsive image -->
<img src="hero-image.jpg" alt="Hero banner" style="max-width: 100%; height: auto;">
<!-- Image with figure and caption -->
<figure>
<img src="chart.png" alt="Sales growth chart">
<figcaption>Company growth over the past year</figcaption>
</figure>
Pro tip: Never skip the alt attribute – it’s essential for accessibility and helps search engines understand your images.
6. List Tags (<ul>, <ol>, <li>)
Whether you’re creating a navigation menu or outlining steps in a process, lists are super handy. You’ve got two main types:
- Unordered lists (
<ul>
) for bullet points - Ordered lists (
<ol>
) for numbered sequences
<!-- Unordered list -->
<ul>
<li>First item</li>
<li>Second item</li>
<li>Third item</li>
</ul>
<!-- Ordered list -->
<ol>
<li>Step one</li>
<li>Step two</li>
<li>Step three</li>
</ol>
<!-- Nested list -->
<ul>
<li>Main item 1
<ul>
<li>Sub item 1.1</li>
<li>Sub item 1.2</li>
</ul>
</li>
<li>Main item 2</li>
</ul>
<!-- Description list -->
<dl>
<dt>HTML</dt>
<dd>HyperText Markup Language</dd>
<dt>CSS</dt>
<dd>Cascading Style Sheets</dd>
</dl>
7. Division Tag (<div>)
The <div>
tag is like a container for grouping elements together. While it doesn’t have any visual effect on its own, it’s perfect for organizing content and applying CSS styles.
<!-- Basic structure -->
<div class="container">
<div class="header">
<h1>Welcome</h1>
</div>
<div class="content">
<p>Main content here</p>
</div>
<div class="footer">
<p>Footer content</p>
</div>
</div>
<!-- Card example -->
<div class="card">
<div class="card-header">
<h3>Product Title</h3>
</div>
<div class="card-body">
<img src="product.jpg" alt="Product image">
<p>Product description</p>
</div>
<div class="card-footer">
<button>Buy Now</button>
</div>
</div>
8. Span Tag (<span>)
Need to style just a few words within a paragraph? The <span>
tag is your go-to inline container. It’s like a <div>
, but for smaller, inline elements.
<p>This is a paragraph with <span style="color: red;">red text</span> in it.</p>
<p>The temperature is <span class="highlight">25°C</span> today.</p>
<p>Status: <span class="badge badge-success">Active</span></p>
<h2>Price: $99 <span class="discount">Save 20%!</span></h2>
9. Form Tags (<form>, <input>)
Forms are how we collect user input. The basic structure looks like this:
<form>
<input type="text" placeholder="Enter your name">
<input type="submit" value="Send">
</form>
There’s a whole world of input types to explore here!
<form action="/submit" method="POST">
<!-- Text input -->
<div class="form-group">
<label for="name">Name:</label>
<input type="text" id="name" name="name" placeholder="Enter your name" required>
</div>
<!-- Email input -->
<div class="form-group">
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
</div>
<!-- Password input -->
<div class="form-group">
<label for="password">Password:</label>
<input type="password" id="password" name="password" minlength="8">
</div>
<!-- Radio buttons -->
<div class="form-group">
<p>Choose your plan:</p>
<input type="radio" id="basic" name="plan" value="basic">
<label for="basic">Basic</label>
<input type="radio" id="premium" name="plan" value="premium">
<label for="premium">Premium</label>
</div>
<!-- Checkbox -->
<div class="form-group">
<input type="checkbox" id="subscribe" name="subscribe">
<label for="subscribe">Subscribe to newsletter</label>
</div>
<!-- Select dropdown -->
<div class="form-group">
<label for="country">Country:</label>
<select id="country" name="country">
<option value="">Select a country</option>
<option value="us">United States</option>
<option value="uk">United Kingdom</option>
<option value="ca">Canada</option>
</select>
</div>
<!-- Textarea -->
<div class="form-group">
<label for="message">Message:</label>
<textarea id="message" name="message" rows="4"></textarea>
</div>
<!-- Submit button -->
<button type="submit">Submit Form</button>
</form>
10. Button Tag (<button>)
Last but not least, the button tag creates clickable buttons for user interaction:
<!-- Basic buttons -->
<button type="button">Click Me</button>
<button type="submit">Submit</button>
<button type="reset">Reset</button>
<!-- Buttons with icons -->
<button type="button">
<img src="icon.svg" alt="">
With Icon
</button>
<!-- Disabled button -->
<button type="button" disabled>Cannot Click</button>
<!-- Button with additional attributes -->
<button
type="button"
class="primary-btn"
onclick="handleClick()"
data-action="save">
Save Changes
</button>
It’s more semantic than using a styled <div>
for clickable elements.
Tables play a vital role in web development for organizing and displaying data effectively, making the <table>
tag one of the key elements every developer should know. If you’re just starting or looking to refine your skills, this Easy Guide to Creating Tables in HTML provides a detailed explanation to help you craft well-structured and visually appealing tables.
Putting It All Together
Here’s an example of how these tags work together:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My Website</title>
</head>
<body>
<div class="container">
<header>
<h1>Welcome to My Website</h1>
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
<li><a href="/contact">Contact</a></li>
</ul>
</nav>
</header>
<main>
<h2>Featured Content</h2>
<div class="card">
<img src="featured.jpg" alt="Featured image">
<h3>Article Title</h3>
<p>This is a brief description with some <span class="highlight">highlighted</span> text.</p>
<button type="button">Read More</button>
</div>
<form class="contact-form">
<h3>Contact Us</h3>
<div class="form-group">
<label for="name">Name:</label>
<input type="text" id="name" required>
</div>
<button type="submit">Send</button>
</form>
</main>
<footer>
<p>© 2024 My Website. All rights reserved.</p>
</footer>
</div>
</body>
</html>
Wrapping Up
These tags are just the beginning! While there are many more HTML elements to learn, mastering these 10 will give you a solid foundation for building web pages. Remember, good HTML is about more than just making things look right – it’s about creating semantic, accessible, and well-structured documents.
Keep practicing, and don’t be afraid to experiment with these tags in different combinations. Before you know it, writing HTML will become second nature!
Happy coding! 💻✨
P.S. Want to level up your HTML game? Start exploring semantic HTML elements like <article>
, <section>
, and <nav>
. They’ll make your code even more meaningful and accessible!